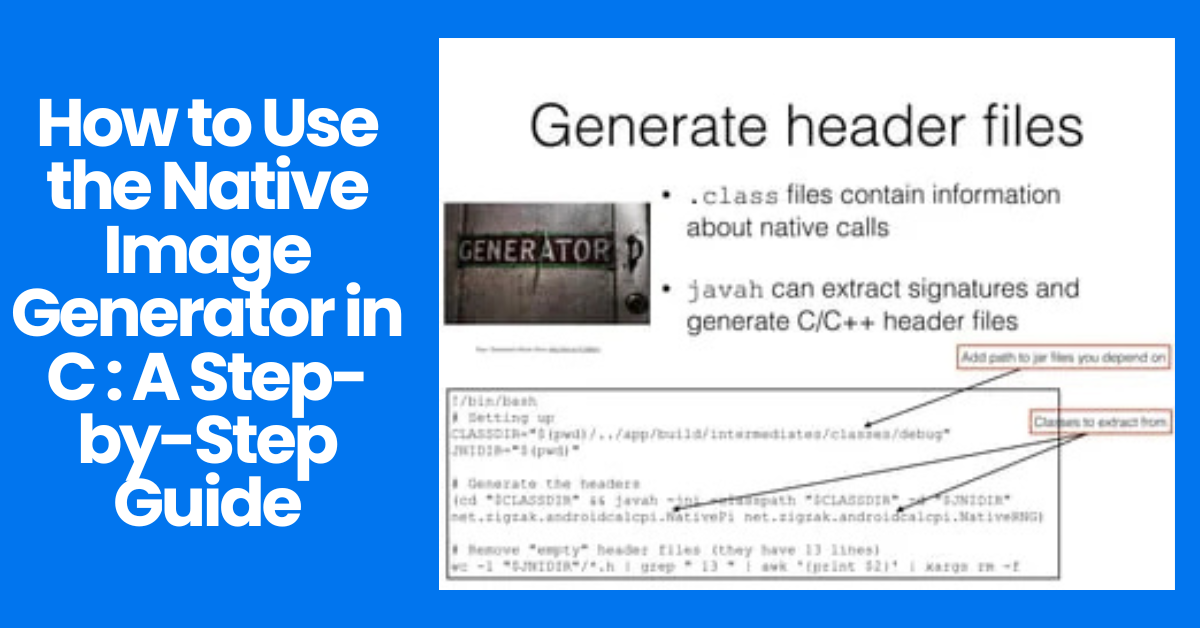
How to Use the Native Image Generator in C: A Step-by-Step Guide
- Image Generators
- November 5, 2024
- No Comments
The world of programming continuously evolves, introducing tools and methodologies that help developers create faster, more efficient applications. One such tool is the Native Image Generator (NIG) in C, which allows developers to compile their applications directly into native machine code, resulting in significant performance improvements. This blog post will serve as a comprehensive guide on how to use the native image generator in C, exploring its setup, functionality, optimization techniques, and future prospects. Whether you are a seasoned developer or just starting, this guide aims to provide valuable insights into leveraging this powerful tool effectively.
How to Use the Native Image Generator in C
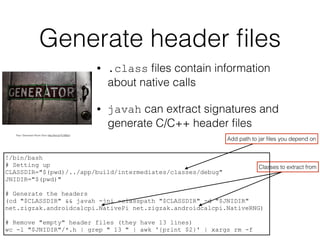
In the realm of software development, the ability to produce optimized native applications is paramount. The Native Image Generator (NIG) is a tool that transforms high-level source code into native machine code, enabling programs to execute with increased speed and reduced overhead.
What is Native Image Generation?
Native image generation refers to the process of converting source code, usually written in high-level languages like C, into binary executable files that can run directly on the hardware without the need for an interpreter or virtual machine. This distinction can drastically enhance performance, particularly for compute-intensive applications that require real-time processing.
The appeal of native images lies not only in their execution speed but also in their ability to reduce startup time for applications. By pre-compiling code into a format that the processor can understand, the application spends less time in the intermediary stages of execution, leading to quicker load times and a more responsive user experience.
Benefits of Using Native Images
Utilizing native images offers several advantages:
- Performance Boost: Native images are typically faster than interpreted or JIT-compiled code, making them ideal for scenarios where performance is critical.
- Reduced Memory Usage: By eliminating the overhead associated with runtime environments, native images can lead to lower memory consumption.
- Enhanced Security: Compiling to native code reduces the attack surface as there are fewer components running during execution.
- Ease of Distribution: Native binaries can be easier to distribute since they do not depend on runtimes being installed on the target machine.
These benefits position native image generation as a compelling option for developers looking to optimize their applications for performance and efficiency.
Setting Up Your Development Environment
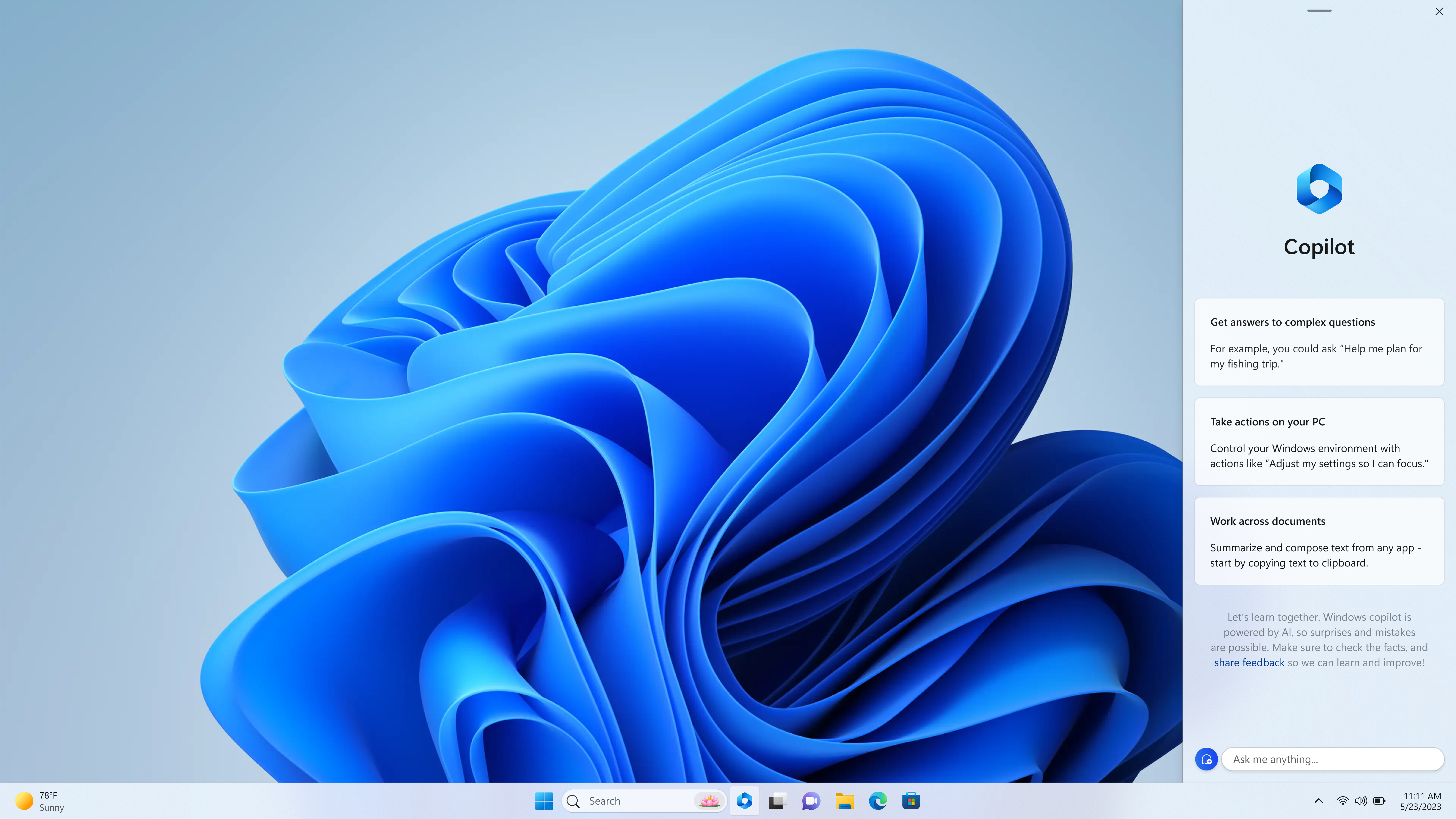
Before diving into the specifics of using the native image generator, it is crucial to set up an appropriate development environment. This involves selecting the right tools, configuring your system, and ensuring all necessary dependencies are in place.
Choosing the Right Compiler
An essential step in setting up your environment is choosing the right compiler. In the context of C programming, popular compilers include GCC (GNU Compiler Collection), Clang, and Microsoft Visual Studio’s compiler. Each compiler has its strengths, and your choice may depend on factors such as platform, specific features, and community support.
For instance, if you’re working on a Unix-like system, GCC is commonly favored due to its extensive documentation and broad acceptance in the open-source community. On the other hand, Clang is known for its modern architecture and excellent error diagnostics, making it a great choice for developers focused on code quality.
Installing Necessary Libraries and Tools
Once you’ve selected a compiler, the next step is to install any necessary libraries or tools that support native image generation. This often includes standard libraries required by your application, as well as any additional packages that might streamline the build process.
Popular libraries include libc
, which is essential for basic C functionality, and other third-party libraries depending on your application’s requirements. Package managers like apt
for Debian-based systems or brew
for macOS can simplify this installation process.
Configuring the Build Environment
After installing the necessary tools and libraries, configuring your build environment is crucial for a seamless development experience. This typically involves creating a build script or makefile that defines compilation rules, managing dependencies, and facilitating testing.
Makefiles allow for automation in compiling and linking your application, and they can be tailored to specify different build configurations (e.g., debug vs release). Ensuring that your environment is organized from the outset can save time and hassle later in the development cycle.
Understanding the Basics of Native Image Generation

To fully appreciate the power of the native image generator, it’s important to understand how it works. This section covers the fundamental concepts behind native image generation, the technologies involved, and how they relate to the overall programming ecosystem.
The Compilation Process Explained
The compilation process in C consists of several key stages: preprocessing, compilation, assembly, and linking. Each stage plays a vital role in transforming your source code into an executable program.
- Preprocessing: During this phase, the preprocessor handles directives such as
include
and#define
, preparing the code for compilation. This stage generates an intermediate file with all macros and included headers expanded. - Compilation: Next comes the actual compilation, where the compiler translates the preprocessed code into assembly language. This is where syntax checks and optimizations occur.
- Assembly: The assembler takes the assembly code and converts it into machine code, producing object files that contain binary representations of functions and data.
- Linking: Finally, the linker combines all the object files together, resolving references between them and producing the final executable. At this point, native image generation can take over, further optimizing this output for performance.
Technologies Behind Native Images
Native image generation leverages various technologies to achieve optimal results. One of the key components is Just-In-Time (JIT) compilation, commonly used in managed runtime environments. While JIT compilation can improve performance dynamically at runtime, it comes with overhead concerning startup time and memory usage.
Alternatively, static compilation produces native images ahead of time. This means that the entire application, including its dependencies, is compiled into a standalone executable, eliminating the need for JIT processes. This shift can significantly enhance performance, especially for long-running applications.
Native Compilation vs Runtime Compilation
It’s important to distinguish between native compilation and runtime compilation. Native compilation occurs once during the build process and produces a fully optimized binary that can run autonomously on compatible hardware.
In contrast, runtime compilation happens on-the-fly while an application is running. Although it offers flexibility, it often incurs performance penalties due to the need for continuous type-checking and interpretation. Understanding these differences is crucial for developers aiming to utilize the native image generator effectively.
Creating a Simple Native Image Application
Having established a foundational understanding of native image generation, let’s explore the steps involved in creating a simple native image application using the native image generator in C.
Writing Your First C Program
Before generating a native image, you must first write a basic C program. Consider a simple “Hello, World!” example to illustrate the process. This code demonstrates function declaration, string handling, and standard input/output operations.
# include
int main() {
printf("Hello, World!\n");
return 0;
}
This straightforward program serves as a perfect starting point, highlighting essential components of C programming while providing a clear path towards native image generation.
Compiling the Program into a Native Image
Once your program is ready, the next step is to compile it into a native image. Using the compiler of your choice, you can employ command-line tools to generate the native binary directly.
For instance, with GCC, the command might look like:
gcc -o hello_world hello_world.c
This command tells GCC to compile hello_world.c
and output an executable named hello_world
. Once executed, this binary can be run independently, demonstrating the advantages of native image generation.
Running Your Native Image Application
After compiling your program, running the generated executable is straightforward. You can invoke it directly from the command line:
./hello_world
Upon execution, you’ll see the output "Hello, World!"
printed to the terminal. This marks your first successful foray into native image generation, showcasing the simplicity and effectiveness of the process.
Exploring the Native Image Generation Process
With a basic understanding and initial experience under your belt, we can delve deeper into the intricacies of the native image generation process itself.
Analyzing the Compilation Output
After compiling your application, it’s beneficial to analyze the compilation output to understand what transpired during the process. Most compilers provide verbose output options, which can reveal insights into the compilation stages, optimization strategies, and potential warnings or errors.
For example, using GCC’s -Wall
flag can help identify common pitfalls in your code:
gcc -Wall -o hello_world hello_world.c
Being able to read and interpret this output is invaluable, as it provides context around the decisions made by the compiler and helps you identify areas for improvement.
Dissecting the Generated Binary
As part of your exploration, you may wish to inspect the generated binary itself. Tools such as objdump
or nm
can display information about the symbols and sections within your binary. This analysis can yield helpful insights regarding memory allocation, function calls, and external dependencies.
For instance, executing objdump -d hello_world
reveals the disassembled machine code, allowing you to examine how the compiler translated your high-level instructions into low-level machine instructions. This layer of understanding is critical for optimizing performance.
Profiling Your Application
Profiling your application using various profiling tools can offer additional insights into its performance characteristics. Tools like gprof
or perf
can provide data on CPU usage, function call frequency, and execution time.
By analyzing this data, you can identify bottlenecks in your code and make informed decisions on optimizations. For instance, if a particular function consumes a disproportionate amount of CPU time, it would warrant a closer inspection to determine how it could be streamlined.
Optimizing for Performance with Native Images
Now that we have a clear understanding of the native image generation process, let’s discuss techniques for optimizing performance when working with native images.
Compiler Optimization Flags
Most compilers support a range of optimization flags that can significantly influence the performance of the generated binary. These flags instruct the compiler on how aggressively to optimize the code, balancing compile time against execution speed.
For example, GCC provides flags such as -O1
, -O2
, and -O3
, each increasing levels of optimization. Employing -O3
enables aggressive optimizations that can result in substantial performance gains, though at the cost of longer compilation times.
gcc -O2 -o hello_world hello_world.c
Using these flags strategically based on your application’s needs can yield impressive results.
Code Refactoring for Efficiency
In addition to utilizing compiler flags, refactoring your code can lead to notable performance enhancements. Techniques such as reducing complexity, minimizing function calls, and avoiding unnecessary computations all contribute to more efficient code.
Consider using algorithms with better asymptotic behavior. For example, if your initial implementation uses a quadratic algorithm to search through a list, switching to a linear or logarithmic alternative can lead to dramatic improvements, particularly for larger datasets.
Memory Management Considerations
Memory management is another critical aspect of performance tuning. Allocating and deallocating memory efficiently can prevent fragmentation and reduce overhead, leading to better speed and responsiveness.
Utilizing stack memory instead of heap memory whenever feasible can provide performance benefits due to the faster allocation/deallocation rates of the stack. Additionally, employing memory pools or custom allocators for frequently-used objects can further enhance performance.
Working with Native Images in Different Contexts
Native images find utility across a variety of application contexts. Understanding how to adapt native image generation techniques to suit specific domains can enhance your skillset significantly.
System Programming
In system programming, where performance and low-level control are paramount, native images can substantially improve efficiency. When developing operating systems, drivers, or embedded systems, leveraging native image generation can lead to faster boot times and more responsive interactions.
Developers often employ native images in kernel modules, where performance requirements are stringent, and minimizing overhead is crucial.
High-Performance Computing (HPC)
The domain of high-performance computing presents a compelling case for native image generation. Applications in scientific computing, simulations, and numerical methods often demand maximum computational efficiency.
By generating native images ahead of time, developers can ensure their applications leverage the full capabilities of the underlying hardware. Techniques such as loop unrolling, vectorization, and parallelization become vital in achieving the desired performance levels.
Web and Cloud Applications
Even in web and cloud applications, native image generation can play a role. With the rise of serverless architectures and microservices, deploying natively compiled executables can enhance cold-start performance and reduce latency.
Frameworks such as AWS Lambda allow developers to deploy native binaries, taking advantage of the quick execution times and minimized resource usage that come with native image generation.
Common Pitfalls and Best Practices
Navigating the landscape of native image generation requires awareness of common pitfalls and best practices. Understanding these challenges can help you avoid costly missteps in your development journey.
Ignoring Platform-Specific Dependencies
One common pitfall developers encounter is neglecting platform-specific dependencies. Native images are not always portable between different operating systems or architectures.
When creating a native image, it’s critical to ensure that all libraries and resources are available on the deployment target. Failing to do so can lead to runtime errors or unexpected behavior when the application is executed.
Over-Optimizing Without Benchmarking
While optimization is essential, it’s equally important to approach it judiciously. Over-optimizing without proper benchmarking can lead to code that is difficult to maintain and understand, while not necessarily providing meaningful performance benefits.
Benchmark your code regularly and focus on optimizing the areas that demonstrate the most considerable performance impact. Tools like Google Benchmark
can assist in establishing performance baselines and tracking the effects of changes over time.
Neglecting Documentation and Maintainability
In the quest for performance, developers sometimes overlook the importance of code readability and maintainability. Native images should be generated from code that remains clear, understandable, and well-documented.
Prioritize writing clean code with descriptive comments and modular design patterns. This practice aids not only in collaboration with others but also in your future self’s efforts to revisit or enhance the code.
Advanced Techniques for Native Image Generation
As you gain confidence in using the native image generator, consider exploring advanced techniques that can further improve your applications’ performance and usability.
Custom Compiler Passes
Developing custom compiler passes can enable targeted optimizations for specific use cases. By extending the compiler’s capabilities, you can implement specialized transformation strategies that align closely with your application’s needs.
This requires a deep understanding of compiler architecture and internals, but the potential rewards—such as tailored performance boosts—can justify the effort.
Utilizing Link Time Optimization (LTO)
Link Time Optimization (LTO) is a technique that allows for optimizations to be performed during the linking stage of the compilation process. By enabling LTO, you can unlock cross-module optimizations, potentially leading to faster execution speeds and reduced binary sizes.
In GCC, you can enable LTO using the -flto
flag:
gcc -o hello_world hello_world.c -flto
Using LTO effectively can elevate your native images’ performance even further by considering the entirety of your application during optimization.
Embracing Cross-Compilation
Cross-compilation expands your development horizons, allowing you to generate native images for different platforms from a single build environment. This capability is particularly advantageous when targeting embedded systems or differing architectures.
Tools like CMake
can facilitate cross-compilation by providing robust support for defining target environments. Familiarizing yourself with cross-compilation will enable you to reach a broader audience with your applications.
The Future of Native Image Generation in C
As technology progresses, so too does the landscape of native image generation. Emerging trends and advancements hold exciting possibilities for developers working within the C programming language.
Integration with Modern Development Practices
With the increasing adoption of agile methodologies and DevOps practices, native image generation is likely to evolve to meet the demands of rapid development cycles. This could entail enhanced tooling for automatic native image generation as part of continuous integration/continuous deployment (CI/CD) pipelines.
Integrating native image generation into CI/CD workflows could streamline the development process, ensuring that optimized binaries are consistently produced and deployed.
Advancements in Machine Learning Optimization
As machine learning techniques continue to advance, integrating intelligent optimization strategies into the native image generation process becomes feasible. Automated analysis of code patterns could lead to recommendations for performance enhancements tailored to each specific application.
Such advancements would empower developers to leverage machine learning insights, driving native image performance to new heights.
Increased Support for Multiple Architectures
With the proliferation of diverse computing architectures—from ARM to RISC-V—support for native image generation across these platforms is likely to grow. As tools and compilers evolve, the ability to generate optimized binaries for various architectures will become more accessible, enhancing cross-platform development capabilities.
Investing in knowledge and skills surrounding multiple architectures will prepare developers to harness the full power of native image generation in the years to come.
Conclusion
Using the native image generator in C opens up a world of possibilities for developers seeking improved performance and efficiency in their applications. From understanding the fundamentals of native image generation to implementing advanced optimization techniques, this guide has equipped you with the knowledge needed to navigate this powerful tool.
As you integrate the native image generator into your development workflow, remember that ongoing learning and adaptation are essential. The landscape of programming continually evolves, presenting new challenges and opportunities. By staying informed and embracing best practices, you can unlock the true potential of native image generation and deliver high-performance applications that stand out in a competitive market.
As you embark on your journey with the native image generator, may your applications achieve unrivaled speed, efficiency, and user satisfaction!
Looking to learn more? Dive into our related article for in-depth insights into the Best Tools For Image Generation. Plus, discover more in our latest blog post on Online Artificial Intelligence Image Generator. Keep exploring with us!
Related Tools:
Image Generation Tools
Video Generators
Productivity Tools
Design Generation Tools
Music Generation Tools